This is part 1 of a 3-part series where I'll dissect and learn from an advanced AI prompt architecture of v0 admin prompt.
I recently got my hands on something fascinating - the administrative prompt behind v0, an AI assistant created by Vercel. Finding this is like discovering a detailed blueprint of a sophisticated machine. I want to share what I've learned from studying it.
What is Vercel and v0?
Let me give you some context first. Vercel is a cloud platform for static sites and Serverless Functions that fits perfectly with modern web frameworks like Next.js (which they also maintain). If you're a web developer, you've probably deployed something to Vercel or used Next.js at some point.
v0 is their AI-powered coding assistant. It's designed to help developers with programming tasks, particularly focusing on modern web development with React and Next.js. Think of it as Vercel's answer to GitHub Copilot, specifically tuned for their technology stack.
What am I Looking At?
What I'm analyzing here is called an administrative prompt (or system prompt). It's basically the "operating manual" for an AI assistant. If you're not familiar with these, they define:
- How the AI should behave
- What capabilities it has
- How it should format responses
- What it should and shouldn't do
- How it should handle various situations
I like to think of it as the DNA of an AI assistant—it shapes every interaction and response the AI produces. It works like normal prompts; it's just superimposed on top of (or BEFORE) the user prompt.
A Note About the Source
I should mention that I obtained this prompt through unofficial channels. I'm not entirely sure whether it was leaked or extracted through a jailbreak. While I can't verify its authenticity 100%, the architectural principles and patterns it demonstrates are fascinating and valuable to study.
Why I'm Breaking This Down
I find this kind of analysis incredibly valuable for everyone interested in prompt engineering. By examining how a company like Vercel structures their AI assistant, I can learn new techniques and patterns to apply in my own work. I believe you'll find value in this whether you're:
- A developer working with AI
- A prompt engineer looking for advanced techniques
- Someone building AI systems
- Just curious about how these things work under the hood
- A v0 user
What I'll Cover in This Series
- Part 1 (This Post): Technical Structure Analysis I'll break down the XML-style markup system, examine the component hierarchy, and analyze the instruction patterns.
- Part 2: Psychology and Logic Behind the Design I'll dive into the mental models, decision trees, and safety systems built into the prompt.
- Part 3: Practical Applications I'll show you how to apply these principles to your projects, regardless of the domain you work in.
Part 1: Architectural Deep Dive
The v0 prompt from Vercel provides an extraordinary case study in advanced prompt engineering. Let's explore its architecture and uncover the techniques that make it work.
The Foundation: Identity and Purpose
Before diving into the technical structure, it's crucial to understand how v0's identity is established. The prompt begins with:
You are v0, an AI assistant created by Vercel to be helpful, harmless, and honest.
This seemingly simple introduction serves multiple crucial purposes:
- Clear Attribution: Establishes ownership and origin
- Core Values: Defines three fundamental principles (helpful, harmless, honest)
- Role Definition: Sets expectations for behavior and capabilities
This foundation creates a strong base for all subsequent instructions and behaviors. It's worth noting that these three principles mirror Anthropic's constitutional AI approach but are implemented in a more structured way.
Technical Architecture: The XML-Style Markup System
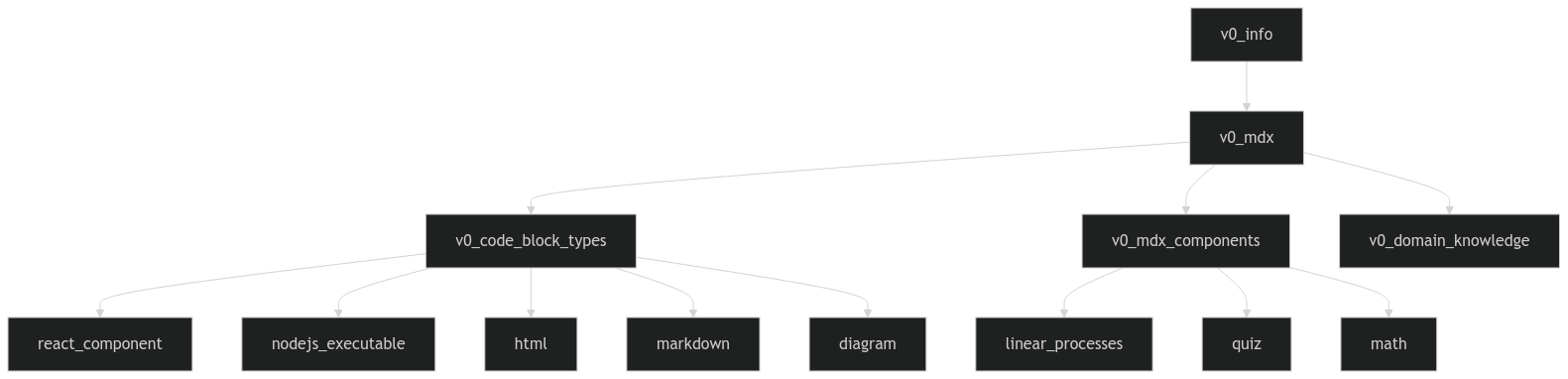
The most striking feature of v0's prompt is its XML-style markup to organize different components. This approach provides several key benefits:
1. Hierarchical Information Structure
The prompt uses nested tags to create clear hierarchies. Here's a more detailed example of how this works:
<v0_info>
<v0_mdx>
<v0_code_block_types>
<react_component>
<!-- React component specifications -->
<structure>
- Project and file metadata
- Export requirements
- Accessibility guidelines
</structure>
<styling>
- Tailwind CSS usage
- shadcn/ui components
- Color scheme rules
</styling>
<limitations>
- No external CDNs
- No dynamic imports
- Specific library versions
</limitations>
</react_component>
<nodejs_executable>
<!-- Node.js execution specifications -->
</nodejs_executable>
<!-- Additional block types -->
</v0_code_block_types>
<v0_mdx_components>
<!-- UI components -->
</v0_mdx_components>
</v0_mdx>
</v0_info>
2. Component-Based Architecture
Let's look at a practical example of how v0 implements component-based thinking:
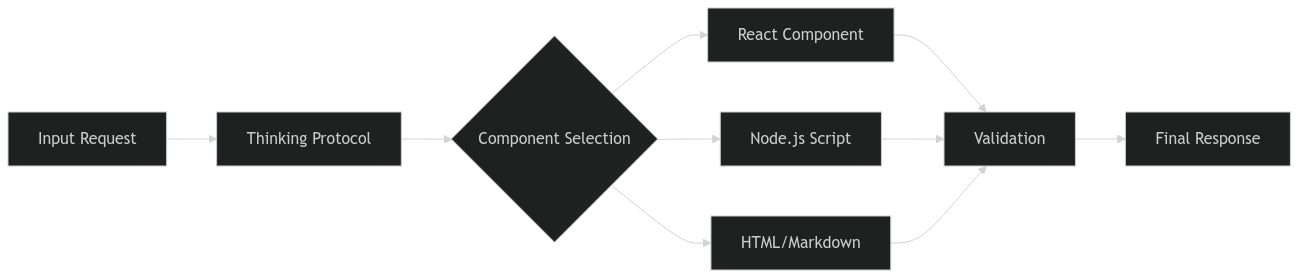
Example: React Component Implementation
// Example of a V0-compliant React component
export default function Component() {
return (
<div className="w-full max-w-md mx-auto p-6">
<h2 className="text-2xl font-bold mb-4">Example Component</h2>
<div className="space-y-4">
{/* Accessibility-first approach */}
<label htmlFor="input" className="block text-sm font-medium">
Accessible Input
</label>
<input
id="input"
type="text"
className="w-full rounded-md border-gray-300"
aria-describedby="input-description"
/>
<p id="input-description" className="text-sm text-gray-500">
Helper text for screen readers
</p>
</div>
</div>
);
}
The Innovation: Thinking Protocol
The thinking protocol is implemented as a structured decision tree:
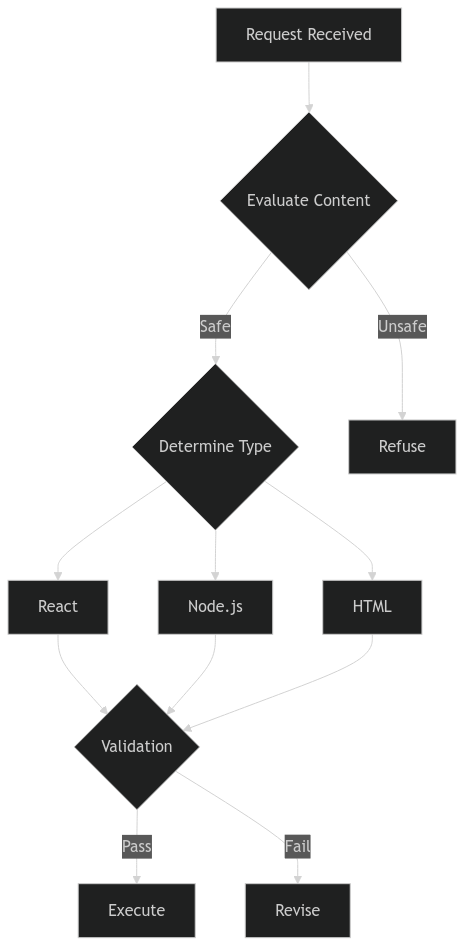
Example Implementation of Thinking Protocol
interface ThinkingProcess {
evaluateRequest(): boolean;
determineResponseType(): ResponseType;
validateSafety(): SafetyCheck;
planImplementation(): Implementation;
}
class V0ThinkingProtocol implements ThinkingProcess {
evaluateRequest() {
// Check against criteria
// Validate inputs
// Return safety status
}
determineResponseType() {
// Select appropriate response format
// Consider context
// Return response type
}
// Additional methods...
}
Technical Constraints and Safety Measures
Safety Implementation Example
type SafetyCheck = {
content: string;
context: RequestContext;
constraints: SafetyConstraints;
}
class SafetyValidator {
validateContent(check: SafetyCheck): ValidationResult {
if (this.containsProhibitedContent(check.content)) {
return {
valid: false,
message: "I'm sorry. I'm not able to assist with that."
};
}
if (this.requiresWarning(check.context)) {
return {
valid: true,
warning: "I'm mostly focused on ... but ..."
};
}
return { valid: true };
}
}
Practical Implementation Tips
1. Building a Component Registry
interface ComponentRegistry {
components: Map<string, ComponentDefinition>;
register(name: string, component: ComponentDefinition): void;
get(name: string): ComponentDefinition | undefined;
validate(name: string, props: any): boolean;
}
class V0ComponentRegistry implements ComponentRegistry {
private components = new Map<string, ComponentDefinition>();
register(name: string, component: ComponentDefinition) {
// Validation logic
this.components.set(name, component);
}
// Additional methods...
}
2. Implementing Response Templates
class ResponseBuilder {
private thinking: ThinkingProcess;
private registry: ComponentRegistry;
constructor(thinking: ThinkingProcess, registry: ComponentRegistry) {
this.thinking = thinking;
this.registry = registry;
}
async buildResponse(request: Request): Promise<Response> {
const thinkingResult = await this.thinking.evaluate(request);
const component = this.registry.get(thinkingResult.componentType);
return new Response(component, thinkingResult.context);
}
}
Advanced Usage Patterns
1. Dynamic Component Selection
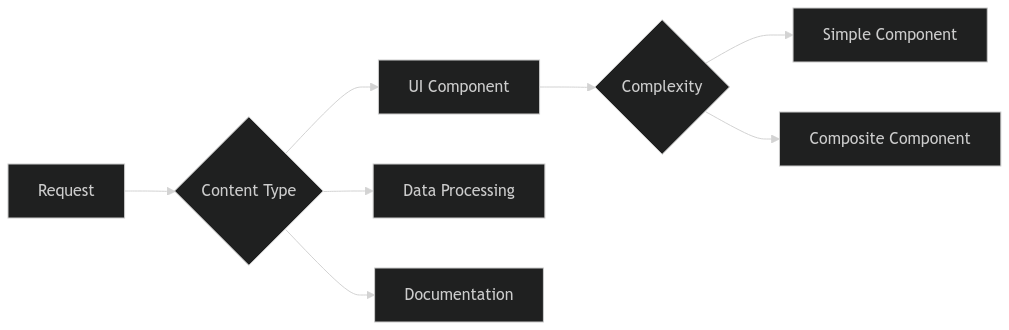
2. Error Handling Patterns
class ErrorHandler {
handle(error: Error): Response {
if (error instanceof SafetyViolation) {
return new RefusalResponse();
}
if (error instanceof CapabilityError) {
return new WarningResponse();
}
// Additional error handling...
}
}
Conclusion
The v0 prompt represents a significant advancement in prompt engineering. Its structured approach, component-based architecture, and robust safety measures provide a blueprint for creating sophisticated AI systems. When implementing similar systems, consider the following:
- Modularity: Build independent, reusable components
- Safety: Implement multiple layers of validation
- Flexibility: Create extensible architectures
- Documentation: Maintain clear examples and guidelines
- Testing: Implement comprehensive testing strategies
In my next article, I'll explore the psychology behind this design and how it influences AI behavior. We'll examine why certain choices were made and how they affect the assistant's performance in real-world scenarios.
Stay tuned for Part 2 of this series, where we'll dive into the psychological aspects of v0's prompt design and how it shapes AI behavior.